Certified Entry-Level Python Programmer Interview Questions
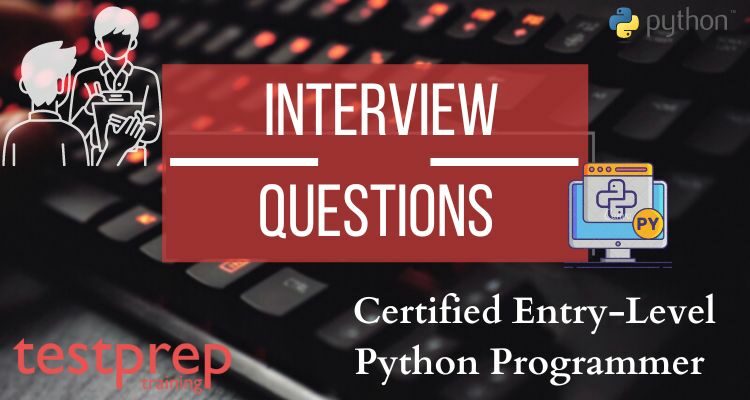
PCEP – Certified Entry-Level Python Programmer accreditation shows that the individual knows about widespread PC programming ideas like information types, compartments, capacities, conditions, circles, as well as Python programming language linguistic structure, semantics, and the runtime climate. Becoming PCEP guaranteed guarantees that the individual is familiar with the most fundamental means given by Python 3 to empower them to begin their own investigations at a middle level and to proceed with their expert turn of events.
Who can take this test?
Here are a few significant requirements to go to this course:
- The individual ought to have more than adequate information on the major ideas of PC programming
- Ought to ready to know the fundamental grammar and semantics of the Python programming language
- The individual is additionally ready to determine run-of-the-mill execution challenges utilizing the Python Standard Library.
1.) What is the Difference Between a Shallow Copy and Deep Copy?
- Deepcopy makes an alternate item and populates it with the youngster objects of the first article. Hence, changes in the first article are not reflected in the duplicate.
copy.deepcopy() makes a Deep Copy.
- Shallow duplicate makes an alternate item and populates it with the references of the kid objects inside the first article. Along these lines, changes in the first article are reflected in the duplicate.
copy.copy makes a Shallow Copy.
2.) How Is Multithreading Achieved in Python?
Multithreading typically infers that various strings are executed simultaneously. The Python Global Interpreter Lock doesn’t permit more than one string to hold the Python translator at that specific mark of time. So multithreading in python is accomplished through setting exchanging. It is very unique in relation to multiprocessing which really opens up numerous cycles across different strings.
3.) Talk about Django Architecture.
Django is a web administration used to fabricate your site pages. Its engineering is as displayed:
- Format: the front finish of the website page
- Model: the back end where the information is put away
- View: It collaborates with the model and format and guides it to the URL
- Django: serves the page to the client
4.) What Advantage Does the Numpy Array Have over a Nested List?
Numpy is written in C so that every one of its intricacies is maneuvered into an easy-to-utilize module. Records, then again, are powerfully composed. Accordingly, Python should check the information sort of every component each time it utilizes it. This makes Numpy exhibits a lot quicker than records.
Numpy has a great deal of extra usefulness that the rundown doesn’t offer; for example, a ton of things can be mechanized in Numpy.
5.) How is Memory overseen in Python?
Python has a private stack space that stores every one of the items. The Python memory administrator controls different parts of this store, like sharing, reserving, division, and designation. The client has zero influence over the stack; just the Python translator approaches.
6.) How Would You Generate Random Numbers in Python?
To produce arbitrary numbers in Python, you should initially import the irregular module.
The irregular() work creates arbitrary float esteem between 0 and 1.
“random.random()”
The randrange() work produces an irregular number inside a given reach.
Language structure: randrange(beginning, end, step)
Model – > random.randrange(1,10,2)
7.) What Does the//Operator Do?
- In Python, the/administrator performs division and returns the remainder in the float.
- For instance: 5/2 returns 2.5
- The//administrator, then again, returns the remainder in whole number.
- For instance: 5//2 brings 2 back
8.) Is Python Object-situated or Functional Programming?
Python is considered a multi-worldview language.
Python follows the article situated worldview
- Python permits the production of articles and their control through explicit techniques
- It upholds a large portion of the highlights of OOPS like legacy and polymorphism
- Python follows the useful programming worldview
Capacities might be utilized as the five-star object
Python upholds Lambda capacities which are normal for the utilitarian worldview
9.) If You Split Your Data into Train/Test Splits, Is It Possible to over Fit Your Model?
Indeed. One normal amateur error is re-tuning a model or preparing new models with various boundaries in the wake of seeing its exhibition on the test set.
10.) Which Python Library Is Built on Top of Matplotlib and Pandas to Ease Data Plotting?
Seaborn is a Python library based on top of matplotlib and pandas to ease information plotting. An information perception library in Python gives an undeniable level connection point to drawing measurable enlightening diagrams.
11.) Clarify Python?
Python is a profoundly complete, intuitive, and object-arranged scriptwriting language. It is explicitly evolved determined to make the substance profoundly intelligible among net surfers. Python utilizes different English catchphrases other than accentuations. It likewise has lesser grammatical developments like in different dialects.
12.) What are the unmistakable elements of Python?
The unmistakable elements of Python incorporate the accompanying.
- Organized and utilitarian projects are upheld.
- It tends to be accumulated to byte-code for making bigger applications.
- Grows significant level powerful information types.
- Upholds checking of dynamic information types.
- Applies computerized trash assortment.
- It very well may be utilized really alongside Java, COBRA, C, C++, ActiveX, and COM.
13.) What is Pythonpath?
A Pythonpath advises the Python translator to find the module records that can be brought into the program. It incorporates the Python source library registry and source code index.
14.) Could we preset Pythonpath?
Indeed, we can preset Pythonpath as a Python installer.
15.) For what reason do we utilize Pythonstartup climate variable?
We utilize the Pythonstartup climate variable since it comprises the way wherein the introduction record conveying Python source code can be executed to begin the mediator.
16.) What is the Pythoncaseok climate variable?
Pythoncaseok climate variable is applied in Windows with the reason to guide Python to track down the main case uncaring match in an import explanation.
17.) What are the upheld standard information types in Python?
The upheld standard information types in Python incorporate the accompanying.
- List.
- Number.
- String.
- Word reference.
- Tuples.
18.) Characterize tuples in Python?
Tuples are an arrangement information type in Python. The quantity of values in tuples is isolated by commas.
19.) What are the positive and negative records?
In the positive lists are applied the hunt creatures from left to the right. On account of the negative lists, the pursuit starts from right to left. For instance, in the exhibit rundown of size n the positive file, the principal record is 0, then, at that point, comes 1, and until the keep going file is n-1. In any case, in the negative list, the main record is – n, then, at that point – (n-1) until the last list will be – 1.
20.) What can be the length of the identifier in Python?
The length of the identifier in Python can be of any length. The longest identifier will abuse from PEP – 8 and PEP – 20.
21.) Characterize Pass explanation in Python?
A Pass explanation in Python is utilized when we can’t choose what to do in our code, however, we should type something for making it linguistically right.
22.) What are the limits of Python?
There are sure restrictions of Python, which incorporate the accompanying:
- It has plan limitations.
- It is slow when contrasted and C and C++ or Java.
- It is wasteful in portable figuring.
- It comprises an immature data set admittance layer.
23.) Do runtime blunders exist in Python? Give a model?
Indeed, runtime mistakes exist in Python. For instance, on the off chance that you are duck composing and things resemble a duck, it is considered as a duck regardless of whether that is only a banner or stamp or some other thing. The code, for this situation, would be A Run-time mistake. For instance, Print “hackr io”, then, at that point, the runtime blunder would be the missing bracket that is expected by print ( ).
24.) Would we be able to turn around a rundown in Python?
Indeed, we can save a rundown in Python utilizing the opposite() technique. The code can be portrayed as follows.
def reverse(s):
str = “”
for i in s:
str = i + str
return str
25.) For what reason do we really want a break in Python?
The break helps in controlling the Python circle by breaking the current circle from execution and moving the control to the following square.
26.) For what reason do we really want a go-on in Python?
A proceed additionally helps in controlling the Python circle yet by taking leaps toward the following emphasis of the circle without debilitating it.
27.) Would we be able to utilize a break and proceed together in Python? How?
Break and proceed can be utilized together in Python. The break will prevent the current circle from execution, while hop will take to another circle.
28.) Does Python uphold a characteristic do-while circle?
No Python doesn’t uphold a characteristic do-while circle.
29.) What number of ways can be applied for applying reverse string?
There are five manners by which they converse string can be applied which incorporate the accompanying.
- Circle
- Recursion
- Stack
- Broadened Slice Syntax
- Switched
30.) What are the various phases of the Life Cycle of a Thread?
The various phases of the Life Cycle of a Thread can be expressed as follows.
Stage 1: Creating a class where we can supersede the run strategy for the Thread class.
Stage 2: We settle on a decision to begin() on the new string. The string is taken forward for planning.
Stage 3: Execution happens wherein the string begins execution, and it arrives at the running state.
Stage 4: Thread delay until the calls to techniques including join() and rest() happens.
Stage 5: After the pausing or execution of the string, the sitting tight string is sent for planning.
Stage 6: Running string is finished by executing the ends and arriving at the dead state.
31.) What is the motivation behind social administrators in Python?
The motivation behind social administrators in Python is to look at values.
32.) What are task administrators in Python?
The task administrators in Python can help in joining every one of the numbers juggling administrators with the task image.
33.) For what reason do we really want enrollment administrators in Python?
We really want enrollment administrators in Python with the reason to affirm assuming the worth is a part in another or not.
35.) How are personality administrators unique in relation to enrollment administrators?
Dissimilar to enrollment administrators, the personality administrators contrast the qualities to see whether they have a similar worth or not.
36.) Portray how multithreading is accomplished in Python.
- Despite the fact that Python accompanies a multi-stringing bundle, in the event that the intention behind multithreading is to speed the code, utilizing the bundle isn’t the go-to choice.
- The bundle has something many refer to as the GIL or Global Interpreter Lock, which is a build. It guarantees that one and only one of the strings execute at some random time. A string procures the GIL and afterward accomplishes work prior to passing it to the following string.
- This happens so quickly that to a client it appears to be that strings are executing in equal. Clearly, this isn’t true as they are simply alternating while at the same time utilizing a similar CPU center. Additionally, GIL passing adds to the general upward to the execution.
- Thus, in the event that you mean to utilize the stringing bundle for accelerating the execution, utilizing the bundle isn’t suggested.
37.) Draw an examination between the reach and xrange in Python.
As far as usefulness, both reach and xrange are indistinguishable. Both consider creating a rundown of numbers. The primary contrast between the two is that while range returns a Python list object, xrange returns an xrange object.
Xrange can’t create a static rundown at runtime the manner in which reach does. Running against the norm, it makes values alongside the necessities through an extraordinary strategy called yielding. It is utilized with a sort of item known as generators.
Assuming that you have an exceptionally tremendous reach for which you really want to produce a rundown, then, at that point, xrange is the capacity to choose. This is particularly important for situations managing a memory-delicate framework, for example, a cell phone.
The reach is a memory monster. Utilizing it requires considerably more memory, particularly assuming the necessity is huge. Thus, making a variety of whole numbers to suit the requirements, can bring about a Memory Error and eventually lead to crashing the program.
38.) Clarify Inheritance and its different sorts in Python?
Legacy empowers a class to procure every one of the individuals from another class. These individuals can be properties, techniques, or both. By giving reusability, legacy makes it simpler to make as well as keep an application.
The class procured is known as the youngster class or the determined class. The one that it gets from is known as the superclass or base class or the parent class. There are 4 types of legacy upheld by Python:
- Single Inheritance – A solitary got class secures from on single superclass.
- Staggered Inheritance – At least 2 different got classes procure from two unmistakable base classes.
- Progressive Inheritance – various kid classes gain from one superclass
- Different Inheritance – A got class gets from a few superclasses.
- Question: Explain how can it be to Get the Google store age of any URL or site page utilizing Python.
- Reply: In request to Get the Google store age of any URL or website page utilizing Python, the accompanying URL design is utilized:
http://webcache.googleusercontent.com/search?q=cache:URLGOESHERE
Essentially supplant URLGOESHERE with the web address of the site or site page whose store you really want to recover and find in Python.
39.) Give a definite clarification about setting up the data set in Django.
The most common way of setting up a data set is started by utilizing the order alter mysite/setting.py. This is a typical Python module with a module-level portrayal of Django settings. Django Depends on SQLite naturally, which is not difficult to be utilized as it requires no other establishment.
SQLite stores information as a solitary document in the filesystem. Presently, you want to advise Django on how to utilize the information base. For this, the task’s setting.py document should be utilized. The following code should be added to the document for making the information base serviceable with the Django project:
Assuming you really want to utilize a data set server other than the SQLite, like MS SQL, MySQL, and PostgreSQL, then, at that point, you want to utilize the information base’s organization apparatuses to make a pristine data set for your Django project.
You need to alter the accompanying keys in the DATABASE ‘default’ thing to make the new information base work with the Django project:
DATABASES = { ‘default’: { ‘ENGINE’ : ‘django.db.backends.sqlite3’, ‘NAME’ : os.path.join(BASE_DIR, ‘db.sqlite3’), } }
Motor – For instance, while working with a MySQL data set supplant ‘django.db.backends.sqlite3’ with ‘django.db.backends.mysql’
NAME – Whether utilizing SQLite or some other data set administration framework, the information base is normally a document on the framework. The NAME ought to contain the full way to the document, including the name of that specific record.
40.) How might you separate between profound duplicate and shallow duplicate?
We utilize a shallow duplicate when another case type gets made. It keeps the qualities that are replicated in the new occurrence. Very much like it duplicates the qualities, the shallow duplicate additionally duplicates the reference pointers.
Reference focuses replicated in the shallow duplicate reference to the first articles. Any progressions made in any individual from the class influence the first duplicate of something very similar. Shallow duplicate empowers quicker execution of the program.
Profound duplicate is utilized for putting away qualities that are as of now replicated. Not at all like shallow duplicate, it doesn’t duplicate the reference pointers to the articles. Profound duplicate makes the reference to an item as well as putting away the new article that is pointed by another item.
Changes made to the first duplicate won’t influence whatever other duplicate that utilizes the referred to or put away item. As opposed to the shallow duplicate, profound duplicate makes execution of a program slow. This is because of the way that it makes a few duplicates for each item that is called.